Mate Execution Software
In the previous article I described a part of the ISA-95 standard; more specifically the process segments. However, process segment are far from the only part that ISA-95 describes. Other parts include: Recipes, Production workflows, ERP-interfacing, Job scheduling, Job monitoring, Employee schedules, Equipment availability, OEE measurements, Equipment Maintenance and many more. The small system that I am making for HolyMate does not need all of these details; as we have no Employees, ERP system or planned schedules. It would also take an unreasonable amount of time to implement from scratch.
What parts of ISA-95 does HolyMate require right now?
My current goal is for every litre of HolyMate to be fully tracable back to its ingredients. This requires a lot of static definitions about the process, which ingredients exist, and what data they generate while producing HolyMate. These static definitions are a large part of ISA-95’s object model. The material models can be seen below:
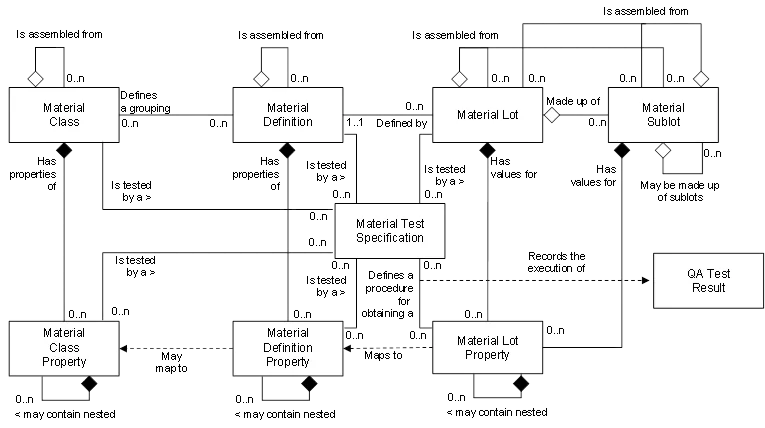
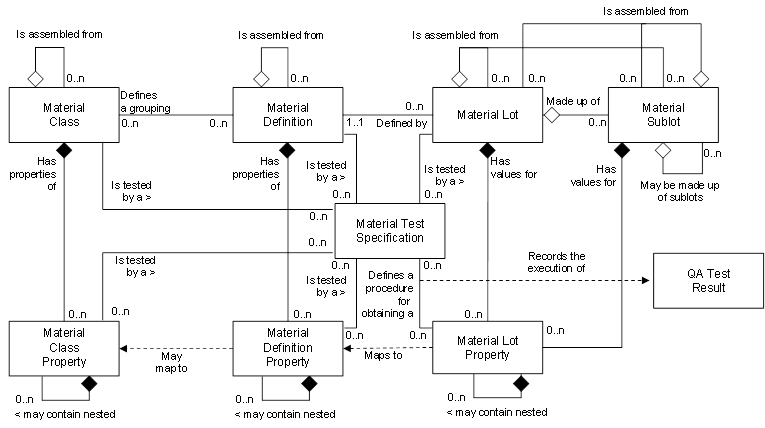
(Image source: OPC Foundation)
Material lots, often physical pallets, are stored somewhere. And these lots are made up out of something, a material that has to be defined somewhere. But if each material gets its own definition that is completely independent; its very hard to define subsitutes, or handle multiple materials which would fall into the same category. That is why the material model exists, it handles material classes, defintions, lots and, if applicable, tests. HolyMate uses ingredients that are stored somewhere, and produces output. To define and track the entire process in the style of ISA-95, the material model is required.
Another part of the ISA-95 model that HolyMate requires is the process model that defines physical locations. This model also allows for a bunch of specifications that can act as overrides for certain recipes, or fill in machine- and location-specifc values. HolyMate does not require any of these overrides (yet), but a definition for process segments is required, because many other models reference it.
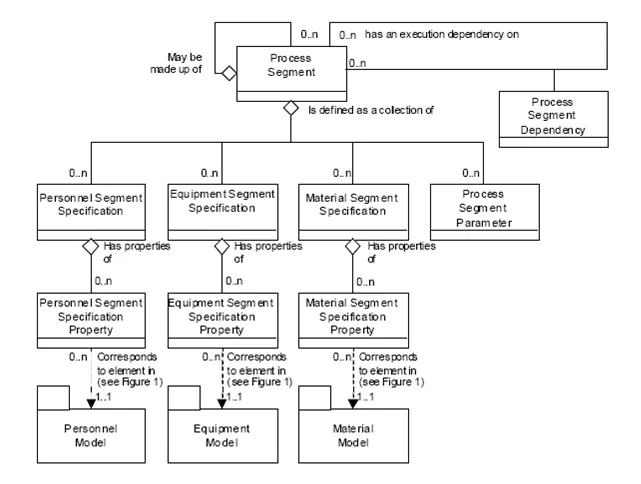
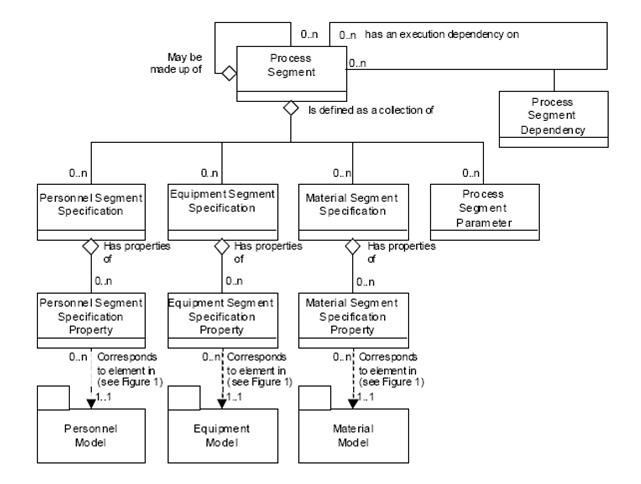
(Image source: Lean validation)
HolyMate requires more models than just these, but this should be enough for the static configuration. Some other models are required for functionality such as scheduling and job tracking.
Implementing these models in C#
Implementing these models in C# is very straight forward, and storing them in a database is equally straight forward when an ORM such as entity framework is used. Simple create a new .NET app, add EntityFramework and GraphQL. Because I am a fan of strong domain models, and all of these models need to be persisted in the (SQL) database, I created the following DbEntry
class which all other domain entities will extend.
namespace HolyMate.MES.Domain;
public class DbEntry
{
public long Id { get; set; }
public bool DbActive { get; set; } = true;
public string Description { get; set; } = "";
public DateTime CreationTime { get; set; } = DateTime.Now;
}
Many models in the ISA-95 standard have properties, which all work in nearly the same way. So I also added a base class which all properties can extend:
public class DbEntryProperty : DbEntry
{
public string Name { get; set; } = "";
public string Value { get; set; } = "";
public string Type { get; set; } = "";
public UnitOfMeasure UnitOfMeasure { get; set; } = UnitOfMeasure.None;
}
This ensures that all tables will use the same base system for Id’s, DbActive’s and meta-data such as a description and creation time. Creating a Material Class is then as simple as the following:
public class MaterialClass : DbEntry
{
public string Name { get; set; } = "";
public ICollection<MaterialClassProperty> Properties { get; set; } = new List<MaterialClassProperty>();
public ICollection<MaterialDefinition> MaterialDefinitions { get; set; } = new List<MaterialDefinition>();
}
public class MaterialClassProperty : DbEntryProperty
{
}
Other models are not much different, simply a public class with a bunch of properties. Entity framework will handle the rest, by generating a bunch of SQL. The generated SQL may not be the most optimal, but its pretty damn good, and I can always override a part if performance becomes a problem. After creating a bunch of simple C# classes, and running the EF generator, DBeaver shows the following ER Diagram:
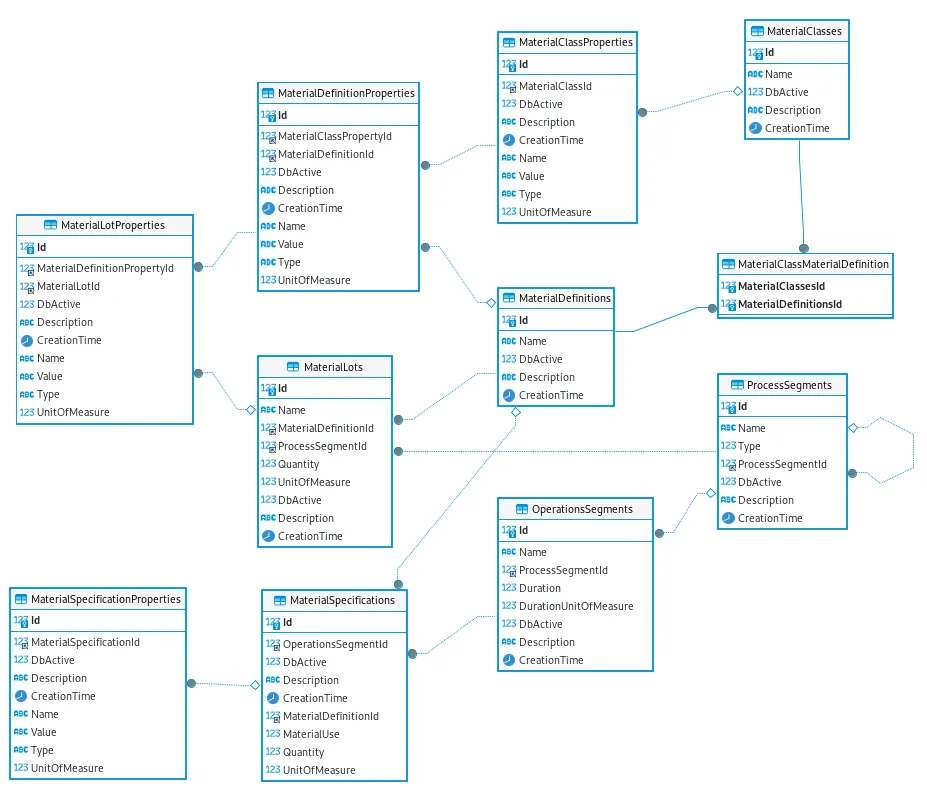
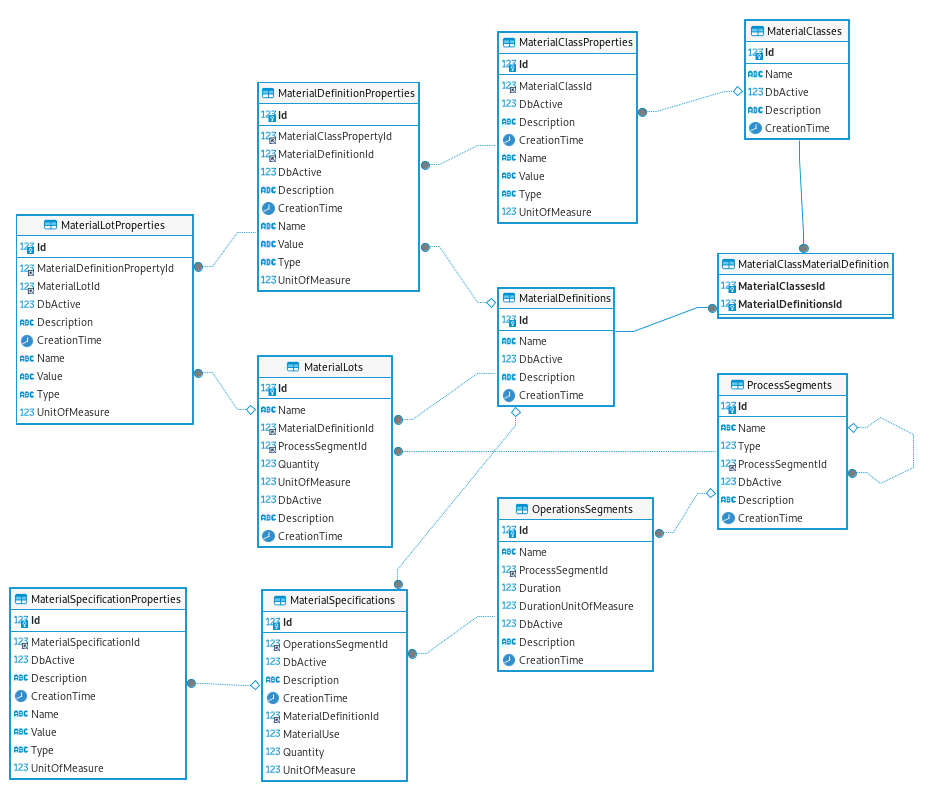
Exposing these models via an API is extremely simple, because I chose to use GraphQL. GraphQL has a very good C# library which can automatically generate a bunch of common API code that allows the use of filtering, sorting, pagination and projection. Projection means that the graphQL query gets translated into a LINQ query that EF can translate into SQL. This means that the server fetches exactly what the API consumer wants from the database, and nothing more. These few lines of code are enough to expose the models via GraphQL:
using HolyMate.MES.Database;
using HolyMate.MES.Domain;
using HolyMate.MES.Domain.ProcessModel;
namespace HolyMate.MES.Query;
public class MesQuery
{
[UseProjection]
[UseFiltering]
[UseSorting]
public IQueryable<MaterialClass> GetMaterialClass(MESContext context) => context.MaterialClasses;
[UseProjection]
[UseFiltering]
[UseSorting]
public IQueryable<MaterialDefinition> GetMaterialDefinition(MESContext context) => context.MaterialDefinitions;
[UseProjection]
[UseFiltering]
[UseSorting]
public IQueryable<ProcessSegment> GetProcessSegment(MESContext context) => context.ProcessSegments;
}
Simply writing a GraphQL query, and firing it towards the API works great. This means that we are ready to create a simple (web) UI that allows the user to define the static environment.
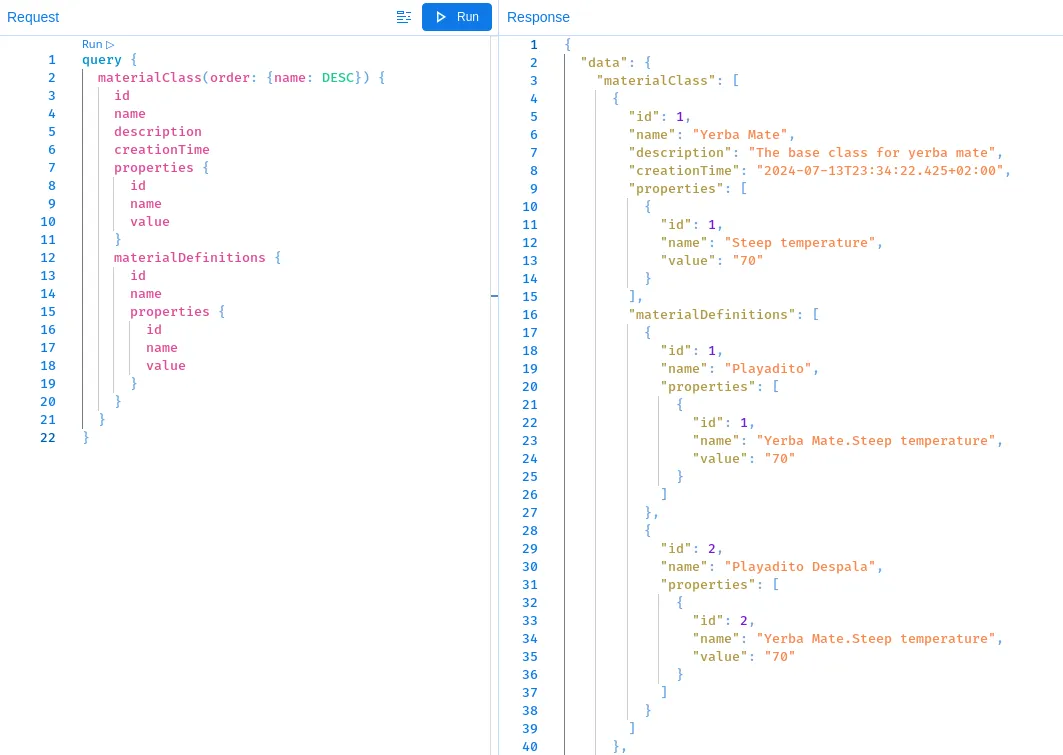
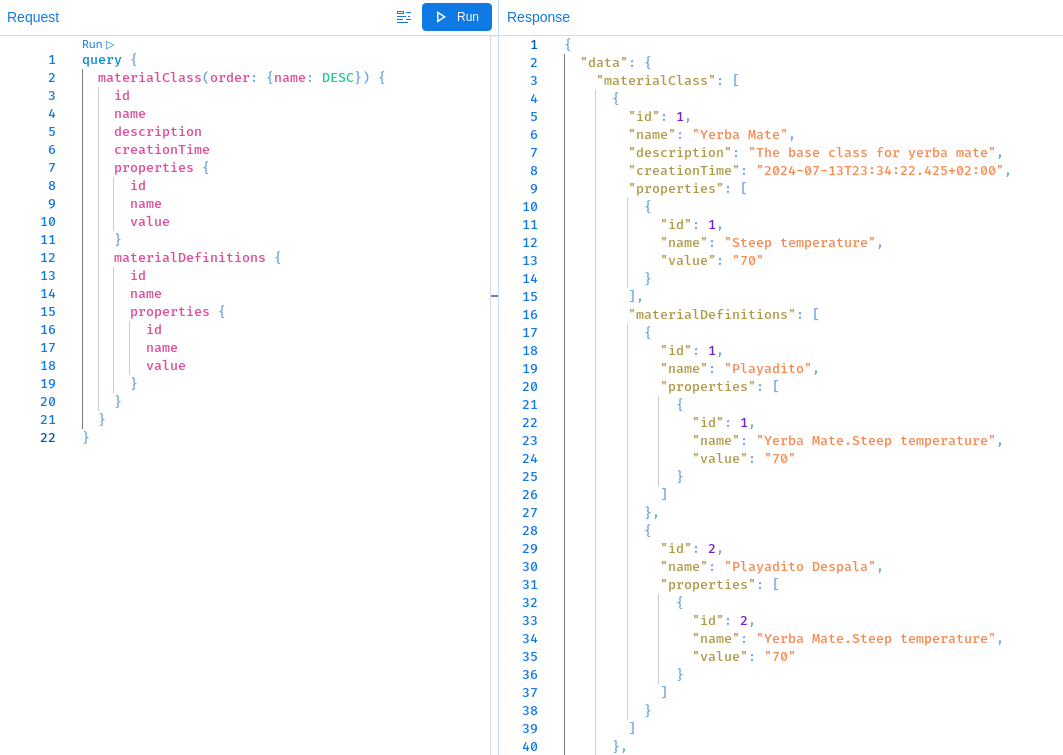
The future
If you have some experience with a web framework such as React, Angular, Vue or whatever else you want; implementing a UI based on top of a GraphQL interface should be fairly trivial. So I did; the work-in-progress version looks like this:
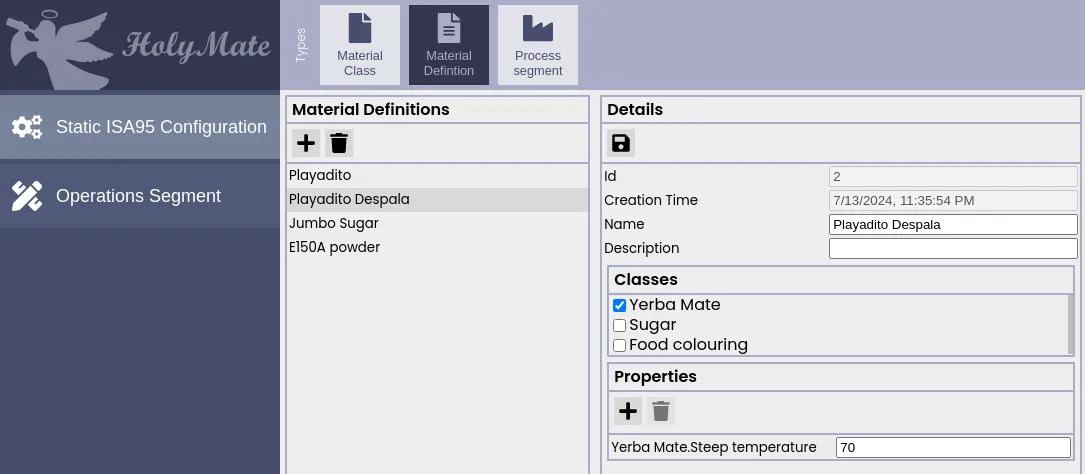
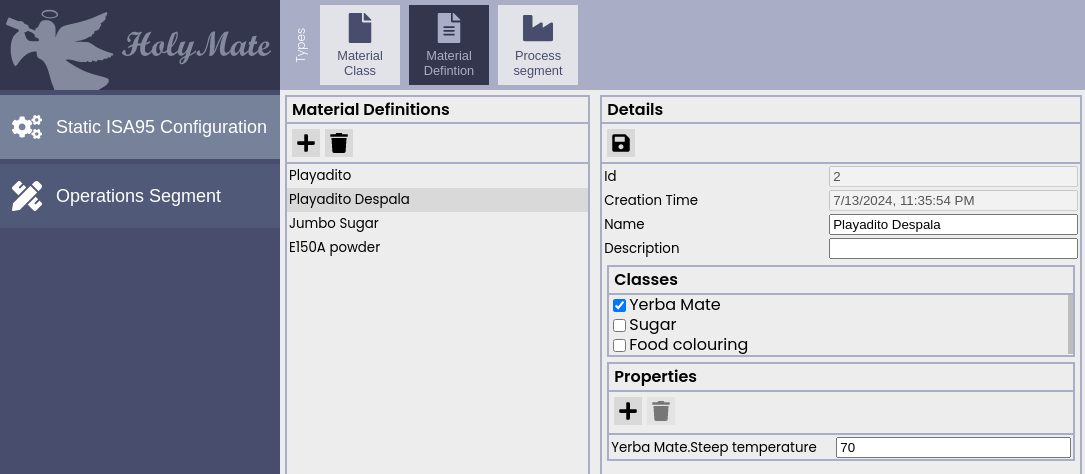
Soon, all required models will be implemented, and the MES system for HolyMate will have the ability to schedule JobOrders, and record data using JobResponses. I also intend to run InfluxDB alongside the SQL database, which will allow for logging of sensor data (such as temperature probes and scales). Most of the system will sadly be locked behind an authentication screen, but I will expose a read-only UI where the user can browse through the data. Imagine being able to enter the code on your HolyMate keg into our website, and it responds with all available production data. That would be sick, and it is coming soon (tm).