The UWU Language
A few weeks ago, I came into contact with peak cringe, the UWU Language ( PDF | Google docs ). This is a made up langage, which can create very simple scentences using a limited vocabulary. I have no clue about the pronounciation of the words, it looks difficult to say the least. The UWU word order is basically english, so if the vocabulary allows it, 1-to-1 translations create readable understandable scentences. An example of this can be seen below:
ow | òvov | uw | üvũv | üvùwu | ũwò |
---|---|---|---|---|---|
I | want | you | to drink | sufficient | water |
The vocabulary is extremely limited, and only has 340 entries, with 868 possible meanings. By contrast, the Oxford English Dictionary contains 273000 entries with 600000 possible meanings. Expressing certain concepts requires some creativity from both the writer and reader. This can be seen in the expression of the word ‘now’ in the following scentence:
ow | úv | ŏwovu | üwów òw |
---|---|---|---|
I | to be ready | death | now (=time current) |
Or the following scentence, where the reader is being asked if they have a headache:
uw | owõv | õvù | öw | úvu | ov? |
---|---|---|---|---|---|
you | to feel | good | with | head | question |
Reading and writing in UWU is tedious, error prone and takes time. This is because all words look similar, and only consist of a handful of letters. That is why I need a tool to help me write and translate UWU. Automatic translation tools are very complex, and take a lot of time to create. There is also the possibility of using a neural network to perform translations, like Deepl does, but this requires tons of training data which does not exist. But 1-to-1 translation created understandable scentences, all that I really needed was an automatic dictionary lookup.
To achieve my goal, I spend some time creating a dictionary in Typescript. This dictionary would allow me to easily perform 1-to-1 translations. In the end, the dictionary looked like this:
export enum WordKind {
Particle,
Adjective,
Adverb,
Numeral,
Conjunction,
Verb,
AuxiliaryVerb,
Pronoun,
Noun,
Preposition,
Demonstrative,
Relative,
}
export interface DictionaryEntry {
word: string;
meanings: { kind: WordKind; desc: string }[];
}
export const Dictionary: DictionaryEntry[] = [
{
word: "nya",
meanings: [{ kind: WordKind.Particle, desc: "if [conditional marker]" }],
},
{
word: "nyáa",
meanings: [{ kind: WordKind.Particle, desc: "please [politeness marker]" }],
},
{
word: "nyanya",
meanings: [{ kind: WordKind.Adjective, desc: "[diminuitive marker]" }],
},
{
word: "o",
meanings: [
{ kind: WordKind.Numeral, desc: "zero" },
{ kind: WordKind.Adverb, desc: "false" },
{ kind: WordKind.Adverb, desc: "no" },
{ kind: WordKind.Adverb, desc: "never" },
],
},
// ...
// The rest is left out for brevity
// Full source: https://git.ironsm4sh.nl/shared/uwuficator
];
When the above dictionary is available, translating uwu to english is essentially as simple as the following:
// Convert input into tokens (split on spaces and remove special marks!)
let output = "";
for (const inputToken of tokens) {
const entry = Dictionary.find((x) => x.word === inputToken);
// TODO: handle dictionary misses
// TODO: handle multiple meanings
output += entry.meanings[0];
}
console.log(output);
However, this still misses the fact that a word can have multiple meanings, and that other features like numbers and special reading marks won’t work. They are left as an exorcise for the reader. The CLI version of my program does this as follows:
$ uwuficator-cli uw owõv õvù öw úvu ov
'you' 'to perceive' 'good' 'with' 'head' 'question'
'to see' 'fine' 'during' 'top'
'to hear' 'fine' 'while' 'upper side'
'to smell' 'well'
'to taste'
'to feel'
'to think'
Where each possible meaning of a word is simply shown on the next line. It is up to the reader to decipher the actual meaning. This works quite well. But this dumb (non-interactive) CLI tool only works when translating from uwu to english, not the other way around. I could make the CLI tool interactive and smarter, but I decided to make it available on the web, users shouldn’t need to install programs that are as simple as this.
The uwuficator is the web version of my tool. The web version can translate from uwu to english, and it can also aid the user in typing scentences in uwu. (Please note that it works better on Chromium based browsers than on Firefox due to the way Firefox presents datalists. This may be resolved in the future)
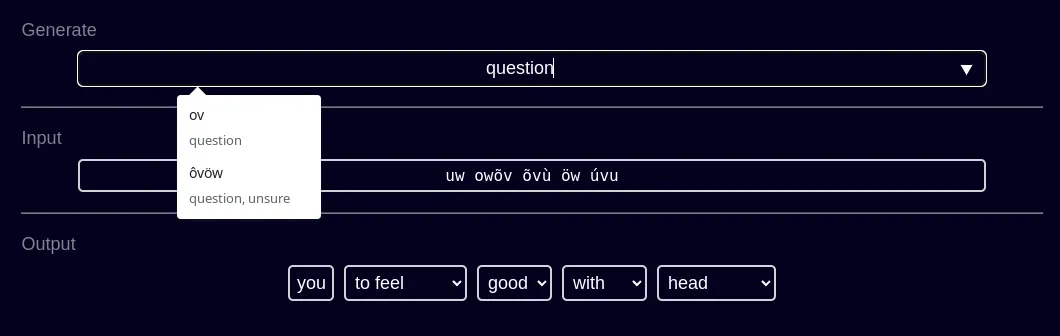
Simply browse/search the dictionary in the top bar, use the arrow keys to select your desired result, and press enter twice to append it to the input. You can also paste an existing uwu scentence into the input, and it will be translated. If a word has multiple meanings, you can browse the meanings using the dropdowns. Full sources of the uwuficator can be found here
Improving the current tool could consist of using an existing AI (such as LLAMA or ChatGPT) to clean up the english output into a correct scentence. But that is currently not implemented. If you have any other ideas that would improve the tools, feel free to contact me!